Man! This has been a pain in the ass since switching routers.
After setting up a DynDNS to point to your IP (in this instance, we'll use "twig.dyndns.com"), this is the ideal workflow from the Internet.
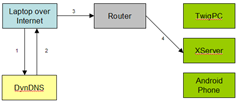
- From outside your home network, access SSH at twig.dyndns.com.
- DynDNS translates the address to your IP "200.100.50.25".
- Client's request reaches your home router
- Which sees the SSH port and forwards to the machine running SSH (XServer)
But for most routers, doing this from the local area network (LAN) has been annoying to say least.
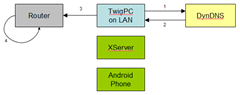
In this instance, we see:
- TwigPC requests access to SSH at "twig.dyndns.com".
- DynDNS translates the address to your internet IP, "200.100.50.25", which is correct.
- TwigPC accesses that IP.
- The router recognises the IP and shortcuts the NAT (network address translation) to itself (Router), rather than forwarding it to the correct device (XServer). Most cases, you'll see access refused or a request timeout.
Here lies the problem called "NAT Loopback" or "NAT Reflection".
Both my Billion 7700N and 5 year old NetComm NB4PlusW had the same issue. Switching to NetGear DGN2000 was good, it resolved the address properly but the damn thing was a nugget.
How to Fix It?
Sorry to say, I haven't found a way to fix this. It's mainly due to the router chipset itself and/or the firmware.
If you DO know of a cure, please let me know!
There are some remedies to relieve the pain, but the main options are...
Edit your hosts file
Method: Direct all traffic for "twig.dyndns.com" to a certain IP within your LAN.
Issues: This will fix the issue for most people, but doesn't really work if you need the "twig.dyndns.com" address to correctly forward depending on port (ie. remote desktop points to TwigPC and SSH points to XServer)
There are already plenty of tutorials on the Internet to show you how to do this for each operating system.
Access it via the LAN host/address
Method: This is easiest for most people. Instead of accessing SSH on "twig.dyndns.org", I just access it via "XServer" instead, bypassing the whole DynDNS issue.
Issues: You'll have to set up your connection profiles twice (once for normal use, once for local area network use).
For example, if I wanted to use Remote Desktop from my Android phone, I need to save 2 connections; one for Internet use (twig.dyndns.com), and then one for LAN use (TwigPC).
Custom Firmware
There are a few alternatives if you're lucky enough to have a router chipset which is supported by a CFW community.
Make sure you check compatibility before doing this!
Sorry.
Sources